This is the third part of a WordPress Gutenberg Blocks series. You can see Part 1 and part 2. The finished repo for this tutorial is located here.
A lot has changed since this article was published a year ago. It’s really hard to keep your writing updated. I think I’ve for the most part succeeded this past year and these articles will be helpful to you. But I want to warn you that there may be updates in dependencies or Gutenberg itself that might need some care if you’re following my examples. I try to write updates as I learn about changes, so also take a look at my most recent posts.
We’ve created a simple block that allows a user to edit an h2 element in a truly WYSIWYG style. We’ve talked about how WordPress Gutenberg does attributes and how that enables us to create interactive elements.
Now, it’s time to include CSS in our block. The Gutenberg editor allows us to enqueue two “new” styles: the editor styles and the view styles. So there are really three stylesheets you should be thinking about when you are developing a block
editor.css | view.css | style.css |
A css file only used in the editor | A css file that is used both in the editor and in the view | The regular styles file that is loaded in a theme for the view only |
Added to WordPress using the enqueue_block_editor_assets hook | Added to WordPress using the enqueue_block_assets hook | Added either by default if you used the default style.css in the theme folder, or by using the function wp_enqueue_style in your theme folder |
Is used specifically to style aspects of the block that deal with editing | Used for styling that should be present for overall layout and presentation | Used for specific theme styles and looks |
Example: When you hover over a picture in the gallery while editing, a “delete this picture” button appears. This a case where it is editor-specific | Example: In a gallery you want the pictures to be laid out 3-up with a 16 px gutter | Example, specific colors, fonts, heading styles, etc… |
And keep in mind that the way I think about it is partially a matter of preference and there are many grey areas. I have heard on many occasions, though that stuff in the theme are for presentation, stuff in a plugin are for functionality. Editor.css and view.css are in the plugin, so technically they should be solely for functionality… (shrug).
Adding Gutenberg block styles through WordPress hooks
In the ‘src’ file add ‘view.css’. Since our “My First RichText Block!” is actually an h2 element, let’s add an h2 style to see that our styles are working:
h2 {
background: orange;
color: white;
}
So now that we have our ugly styles, how do we let WordPress know to include them? We return to our firstgutyblocks.php file and enqueue the file. Add the following to the bottom of this file:
function firstgutyblocks_hello_world_assets() {
wp_enqueue_style(
'firstgutyblocks/hello-world',
plugins_url( 'src/view.css', __FILE__ ),
array( 'wp-edit-blocks' )
);
}
add_action( 'enqueue_block_assets', 'firstgutyblocks_hello_world_assets' );
Now you should see those styles in the editor and the view. But yet again, there’s another problem!
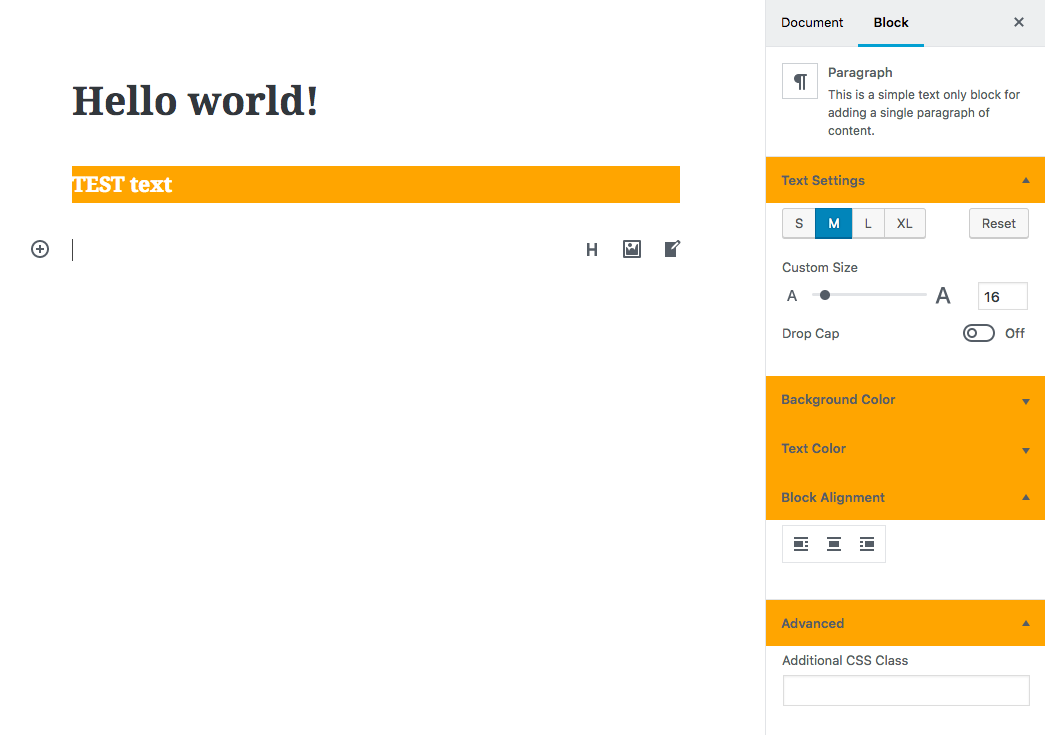
We are affecting things outside of our block because our CSS is not targeting our block class. So let’s talk about how to handle classes in our Gutenberg Block markup.
Controlling class names with Gutenberg Blocks
If you inspect our block as it is, there actually is a class being automatically applied to our block:

Gutenberg will automatically place ‘wp-block-{name used to register block in js}’ on the containing element. This is all well and good if we want to target just that outer container, so let’s change our css:
.wp-block-firstgutyblocks-hello-world {
background: orange;
color: white;
}
Now, we should see the styles being only applied to our block. Sweet, sweet victory.
But, let’s say, you wanted to have an h2 inside of a div, and that outer div had the classname? We can start manually placing the classname by changing our editing and saving methods. The key is that Gutenberg gives you the block class name in the props it send edit() and save(). The prop you will now use is “className”
edit(props) {
const {
setAttributes,
attributes,
className // The class name as a string!
} = props;
function onTextChange(changes) {
setAttributes({
textString: changes
});
}
return (
// We've added a container div
// and we're placing our styles on that manually
<div className={className}>
<RichText
tagName="h2"
value={attributes.textString}
onChange={onTextChange}
placeholder="Enter your text here!"
/>
</div>
);
},
save(props) {
const { attributes, className } = props;
return (
<div className={className}>
<h2>{attributes.textString}</h2>
</div>
);
}
One thing is different now, though. If you load up the block in the editor again, you will have an orange background but black text. If you update, you will have an orange background and white text.
The reason for this is that you aren’t targeting the h2 color specifically, and the Gutenberg editor has styles that color headings black.
So here’s a suggestion for how to fix that:
.wp-block-firstgutyblocks-hello-world {
background: orange;
}
.wp-block-firstgutyblocks-hello-world h2 {
color: white;
}
Enqueueing editor-specific styles
The stylesheet we just added will always be used in the editor and in the view. Sometimes, though, there will be elements that are used in the editor only that need to be styled. So how to we enqueue those stylesheets?
Let’s create a stylesheet named ‘editor.css’ with the following:
.wp-block-firstgutyblocks-hello-world {
position: relative;
}
.wp-block-firstgutyblocks-hello-world:before {
position: absolute;
top: 0;
right: 1em;
content: 'Editor mode!';
color: navy;
font-weight: bolder;
}
This will place a pseudo-element only when we’re editing the block. In our php file we will also have to edit firstgutyblocks_hello_world_editor_assests():
function firstgutyblocks_hello_world_editor_assets() {
wp_enqueue_script(
'firstgutyblocks/hello-world',
plugins_url( 'build/index.build.js', __FILE__ ),
array( 'wp-blocks', 'wp-element' )
);
wp_enqueue_style(
'firstgutyblocks/hello-world-editor-style',
plugins_url( 'src/editor.css', __FILE__ ),
array( 'wp-edit-blocks' )
);
};
add_action( 'enqueue_block_editor_assets', 'firstgutyblocks_hello_world_editor_assets');
It is important to name wp_enqueue_style differently (in our case ‘hello-world-editor-style’) to make sure there aren’t conflicts in enqueuing. Also, be careful to change the dependency from ‘wp-blocks’ to ‘wp-edit-blocks’.
Hopefully you will see:
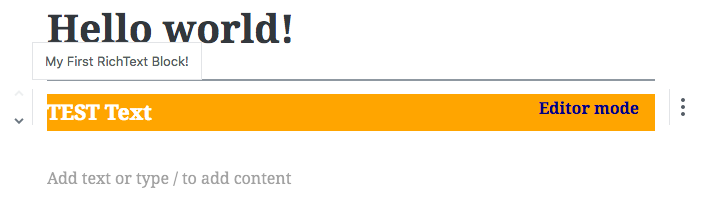
So when is this useful? I’ve used it a couple times where elements I created elments that only appear in the editor. For example, when I created a gallery, I had buttons that allowed the editor to move images left/right or remove them. These buttons need some sort of love and styling, but only occur in the editor.
Recap
We’ve gone over how to enqueue view and editor stylesheets. We also used the className prop to manually place classNames within our blocks.
Next up we need to look at RichText more in depth and include InspectorControls and an editing Toolbar.
Thank you very much for these tutorials … There is not much on this theme. I did part 1, 2 and 3. I will continue with part 4 and 5.
Thank you for the info! One problem I have is how to push a CSS update for a block without deleting the block / re-adding it. Do you know the best method of updating CSS for a block that’s already in use?
I think really the best way is to look at the classes of the block and writing specific CSS in your normal styles location.
You might be able to register styles for existing blocks, but you might have to research that.